Migrate `@FfiNative` to `@Native` (#39034)
diff --git a/lib/ui/compositing.dart b/lib/ui/compositing.dart
index 2c2c6dd..a55480c 100644
--- a/lib/ui/compositing.dart
+++ b/lib/ui/compositing.dart
@@ -34,7 +34,7 @@
return Image._(image, image.width, image.height);
}
- @FfiNative<Handle Function(Pointer<Void>, Uint32, Uint32, Handle)>('Scene::toImageSync')
+ @Native<Handle Function(Pointer<Void>, Uint32, Uint32, Handle)>(symbol: 'Scene::toImageSync')
external String? _toImageSync(int width, int height, _Image outImage);
/// Creates a raster image representation of the current state of the scene.
@@ -58,7 +58,7 @@
);
}
- @FfiNative<Handle Function(Pointer<Void>, Uint32, Uint32, Handle)>('Scene::toImage')
+ @Native<Handle Function(Pointer<Void>, Uint32, Uint32, Handle)>(symbol: 'Scene::toImage')
external String? _toImage(int width, int height, _Callback<_Image?> callback);
/// Releases the resources used by this scene.
@@ -67,7 +67,7 @@
///
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('Scene::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Scene::dispose')
external void dispose();
}
@@ -235,7 +235,7 @@
_constructor();
}
- @FfiNative<Void Function(Handle)>('SceneBuilder::Create')
+ @Native<Void Function(Handle)>(symbol: 'SceneBuilder::Create')
external void _constructor();
// Layers used in this scene.
@@ -332,7 +332,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle, Handle)>('SceneBuilder::pushTransformHandle')
+ @Native<Void Function(Pointer<Void>, Handle, Handle, Handle)>(symbol: 'SceneBuilder::pushTransformHandle')
external void _pushTransform(EngineLayer layer, Float64List matrix4, EngineLayer? oldLayer);
/// Pushes an offset operation onto the operation stack.
@@ -357,7 +357,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Double, Double, Handle)>('SceneBuilder::pushOffset')
+ @Native<Void Function(Pointer<Void>, Handle, Double, Double, Handle)>(symbol: 'SceneBuilder::pushOffset')
external void _pushOffset(EngineLayer layer, double dx, double dy, EngineLayer? oldLayer);
/// Pushes a rectangular clip operation onto the operation stack.
@@ -386,7 +386,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Double, Double, Double, Double, Int32, Handle)>('SceneBuilder::pushClipRect')
+ @Native<Void Function(Pointer<Void>, Handle, Double, Double, Double, Double, Int32, Handle)>(symbol: 'SceneBuilder::pushClipRect')
external void _pushClipRect(
EngineLayer outEngineLayer,
double left,
@@ -421,7 +421,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle, Int32, Handle)>('SceneBuilder::pushClipRRect')
+ @Native<Void Function(Pointer<Void>, Handle, Handle, Int32, Handle)>(symbol: 'SceneBuilder::pushClipRRect')
external void _pushClipRRect(EngineLayer layer, Float32List rrect, int clipBehavior, EngineLayer? oldLayer);
/// Pushes a path clip operation onto the operation stack.
@@ -449,7 +449,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Pointer<Void>, Int32, Handle)>('SceneBuilder::pushClipPath')
+ @Native<Void Function(Pointer<Void>, Handle, Pointer<Void>, Int32, Handle)>(symbol: 'SceneBuilder::pushClipPath')
external void _pushClipPath(EngineLayer layer, Path path, int clipBehavior, EngineLayer? oldLayer);
/// Pushes an opacity operation onto the operation stack.
@@ -477,7 +477,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Int32, Double, Double, Handle)>('SceneBuilder::pushOpacity')
+ @Native<Void Function(Pointer<Void>, Handle, Int32, Double, Double, Handle)>(symbol: 'SceneBuilder::pushOpacity')
external void _pushOpacity(EngineLayer layer, int alpha, double dx, double dy, EngineLayer? oldLayer);
/// Pushes a color filter operation onto the operation stack.
@@ -505,7 +505,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Pointer<Void>, Handle)>('SceneBuilder::pushColorFilter')
+ @Native<Void Function(Pointer<Void>, Handle, Pointer<Void>, Handle)>(symbol: 'SceneBuilder::pushColorFilter')
external void _pushColorFilter(EngineLayer layer, _ColorFilter filter, EngineLayer? oldLayer);
/// Pushes an image filter operation onto the operation stack.
@@ -534,7 +534,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Pointer<Void>, Double, Double, Handle)>('SceneBuilder::pushImageFilter')
+ @Native<Void Function(Pointer<Void>, Handle, Pointer<Void>, Double, Double, Handle)>(symbol: 'SceneBuilder::pushImageFilter')
external void _pushImageFilter(EngineLayer outEngineLayer, _ImageFilter filter, double dx, double dy, EngineLayer? oldLayer);
/// Pushes a backdrop filter operation onto the operation stack.
@@ -561,7 +561,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Pointer<Void>, Int32, Handle)>('SceneBuilder::pushBackdropFilter')
+ @Native<Void Function(Pointer<Void>, Handle, Pointer<Void>, Int32, Handle)>(symbol: 'SceneBuilder::pushBackdropFilter')
external void _pushBackdropFilter(EngineLayer outEngineLayer, _ImageFilter filter, int blendMode, EngineLayer? oldLayer);
/// Pushes a shader mask operation onto the operation stack.
@@ -599,8 +599,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Pointer<Void>, Double, Double, Double, Double, Int32, Int32, Handle)>(
- 'SceneBuilder::pushShaderMask')
+ @Native<Void Function(Pointer<Void>, Handle, Pointer<Void>, Double, Double, Double, Double, Int32, Int32, Handle)>(symbol: 'SceneBuilder::pushShaderMask')
external void _pushShaderMask(
EngineLayer engineLayer,
Shader shader,
@@ -649,7 +648,7 @@
return layer;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Pointer<Void>, Double, Int32, Int32, Int32, Handle)>('SceneBuilder::pushPhysicalShape')
+ @Native<Void Function(Pointer<Void>, Handle, Pointer<Void>, Double, Int32, Int32, Int32, Handle)>(symbol: 'SceneBuilder::pushPhysicalShape')
external void _pushPhysicalShape(
EngineLayer outEngineLayer,
Path path,
@@ -672,7 +671,7 @@
_pop();
}
- @FfiNative<Void Function(Pointer<Void>)>('SceneBuilder::pop', isLeaf: true)
+ @Native<Void Function(Pointer<Void>)>(symbol: 'SceneBuilder::pop', isLeaf: true)
external void _pop();
/// Add a retained engine layer subtree from previous frames.
@@ -712,7 +711,7 @@
_addRetained(wrapper._nativeLayer!);
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('SceneBuilder::addRetained')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'SceneBuilder::addRetained')
external void _addRetained(EngineLayer retainedLayer);
/// Adds an object to the scene that displays performance statistics.
@@ -743,7 +742,7 @@
_addPerformanceOverlay(enabledOptions, bounds.left, bounds.right, bounds.top, bounds.bottom);
}
- @FfiNative<Void Function(Pointer<Void>, Uint64, Double, Double, Double, Double)>('SceneBuilder::addPerformanceOverlay', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Uint64, Double, Double, Double, Double)>(symbol: 'SceneBuilder::addPerformanceOverlay', isLeaf: true)
external void _addPerformanceOverlay(int enabledOptions, double left, double right, double top, double bottom);
/// Adds a [Picture] to the scene.
@@ -778,7 +777,7 @@
_addPicture(offset.dx, offset.dy, picture, hints);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Pointer<Void>, Int32)>('SceneBuilder::addPicture')
+ @Native<Void Function(Pointer<Void>, Double, Double, Pointer<Void>, Int32)>(symbol: 'SceneBuilder::addPicture')
external void _addPicture(double dx, double dy, Picture picture, int hints);
/// Adds a backend texture to the scene.
@@ -804,7 +803,7 @@
_addTexture(offset.dx, offset.dy, width, height, textureId, freeze, filterQuality.index);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Double, Int64, Bool, Int32)>('SceneBuilder::addTexture', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Int64, Bool, Int32)>(symbol: 'SceneBuilder::addTexture', isLeaf: true)
external void _addTexture(double dx, double dy, double width, double height, int textureId, bool freeze, int filterQuality);
/// Adds a platform view (e.g an iOS UIView) to the scene.
@@ -833,7 +832,7 @@
_addPlatformView(offset.dx, offset.dy, width, height, viewId);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Double, Int64)>('SceneBuilder::addPlatformView', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Int64)>(symbol: 'SceneBuilder::addPlatformView', isLeaf: true)
external void _addPlatformView(double dx, double dy, double width, double height, int viewId);
/// Sets a threshold after which additional debugging information should be recorded.
@@ -842,7 +841,7 @@
/// interested in using this feature, please contact [flutter-dev](https://groups.google.com/forum/#!forum/flutter-dev).
/// We'll hopefully be able to figure out how to make this feature more useful
/// to you.
- @FfiNative<Void Function(Pointer<Void>, Uint32)>('SceneBuilder::setRasterizerTracingThreshold', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Uint32)>(symbol: 'SceneBuilder::setRasterizerTracingThreshold', isLeaf: true)
external void setRasterizerTracingThreshold(int frameInterval);
/// Sets whether the raster cache should checkerboard cached entries. This is
@@ -860,14 +859,14 @@
///
/// Currently this interface is difficult to use by end-developers. If you're
/// interested in using this feature, please contact [flutter-dev](https://groups.google.com/forum/#!forum/flutter-dev).
- @FfiNative<Void Function(Pointer<Void>, Bool)>('SceneBuilder::setCheckerboardRasterCacheImages', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Bool)>(symbol: 'SceneBuilder::setCheckerboardRasterCacheImages', isLeaf: true)
external void setCheckerboardRasterCacheImages(bool checkerboard);
/// Sets whether the compositor should checkerboard layers that are rendered
/// to offscreen bitmaps.
///
/// This is only useful for debugging purposes.
- @FfiNative<Void Function(Pointer<Void>, Bool)>('SceneBuilder::setCheckerboardOffscreenLayers', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Bool)>(symbol: 'SceneBuilder::setCheckerboardOffscreenLayers', isLeaf: true)
external void setCheckerboardOffscreenLayers(bool checkerboard);
/// Finishes building the scene.
@@ -884,6 +883,6 @@
return scene;
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('SceneBuilder::build')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'SceneBuilder::build')
external void _build(Scene outScene);
}
diff --git a/lib/ui/dart_ui.cc b/lib/ui/dart_ui.cc
index 24af045..8d14a2f 100644
--- a/lib/ui/dart_ui.cc
+++ b/lib/ui/dart_ui.cc
@@ -55,7 +55,7 @@
typedef CanvasGradient Gradient;
typedef CanvasPath Path;
-// List of native static functions used as @FfiNative functions.
+// List of native static functions used as @Native functions.
// Items are tuples of ('function_name', 'parameter_count'), where:
// 'function_name' is the fully qualified name of the native function.
// 'parameter_count' is the number of parameters the function has.
@@ -65,8 +65,8 @@
// bindings.
// If the name does not match a native function, the template will fail to
// instatiate, resulting in a compile time error.
-// - Resolve the native function pointer associated with an @FfiNative function.
-// If there is a mismatch between name or parameter count an @FfiNative is
+// - Resolve the native function pointer associated with an @Native function.
+// If there is a mismatch between name or parameter count an @Native is
// trying to resolve, an exception will be thrown.
#define FFI_FUNCTION_LIST(V) \
/* Constructors */ \
@@ -117,7 +117,7 @@
V(DartPluginRegistrant_EnsureInitialized, 0) \
V(Vertices::init, 6)
-// List of native instance methods used as @FfiNative functions.
+// List of native instance methods used as @Native functions.
// Items are tuples of ('class_name', 'method_name', 'parameter_count'), where:
// 'class_name' is the name of the class containing the method.
// 'method_name' is the name of the method.
@@ -129,8 +129,8 @@
// bindings.
// If the name does not match a native function, the template will fail to
// instatiate, resulting in a compile time error.
-// - Resolve the native function pointer associated with an @FfiNative function.
-// If there is a mismatch between names or parameter count an @FfiNative is
+// - Resolve the native function pointer associated with an @Native function.
+// If there is a mismatch between names or parameter count an @Native is
// trying to resolve, an exception will be thrown.
#define FFI_METHOD_LIST(V) \
V(Canvas, clipPath, 3) \
diff --git a/lib/ui/experiments/scene.dart b/lib/ui/experiments/scene.dart
index 73e689b..3f2b242 100644
--- a/lib/ui/experiments/scene.dart
+++ b/lib/ui/experiments/scene.dart
@@ -105,25 +105,25 @@
});
}
- @FfiNative<Void Function(Handle)>('SceneNode::Create')
+ @Native<Void Function(Handle)>(symbol: 'SceneNode::Create')
external void _constructor();
- @FfiNative<Handle Function(Pointer<Void>, Handle, Handle)>('SceneNode::initFromAsset')
+ @Native<Handle Function(Pointer<Void>, Handle, Handle)>(symbol: 'SceneNode::initFromAsset')
external String _initFromAsset(String assetKey, _Callback<void> completionCallback);
- @FfiNative<Void Function(Pointer<Void>, Handle)>('SceneNode::initFromTransform')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'SceneNode::initFromTransform')
external void _initFromTransform(Float64List matrix4);
- @FfiNative<Void Function(Pointer<Void>, Handle)>('SceneNode::AddChild')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'SceneNode::AddChild')
external void _addChild(SceneNode sceneNode);
- @FfiNative<Void Function(Pointer<Void>, Handle)>('SceneNode::SetTransform')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'SceneNode::SetTransform')
external void _setTransform(Float64List matrix4);
- @FfiNative<Void Function(Pointer<Void>, Handle, Bool, Bool, Double, Double)>('SceneNode::SetAnimationState')
+ @Native<Void Function(Pointer<Void>, Handle, Bool, Bool, Double, Double)>(symbol: 'SceneNode::SetAnimationState')
external void _setAnimationState(String animationName, bool playing, bool loop, double weight, double timeScale);
- @FfiNative<Void Function(Pointer<Void>, Handle, Double)>('SceneNode::SeekAnimation')
+ @Native<Void Function(Pointer<Void>, Handle, Double)>(symbol: 'SceneNode::SeekAnimation')
external void _seekAnimation(String animationName, double time);
/// Returns a fresh instance of [SceneShader].
@@ -203,12 +203,12 @@
_dispose();
}
- @FfiNative<Void Function(Handle, Handle)>('SceneShader::Create')
+ @Native<Void Function(Handle, Handle)>(symbol: 'SceneShader::Create')
external void _constructor(SceneNode node);
- @FfiNative<Void Function(Pointer<Void>, Handle)>('SceneShader::SetCameraTransform')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'SceneShader::SetCameraTransform')
external void _setCameraTransform(Float64List matrix4);
- @FfiNative<Void Function(Pointer<Void>)>('SceneShader::Dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'SceneShader::Dispose')
external void _dispose();
}
diff --git a/lib/ui/isolate_name_server.dart b/lib/ui/isolate_name_server.dart
index d19012f..d2b2f29 100644
--- a/lib/ui/isolate_name_server.dart
+++ b/lib/ui/isolate_name_server.dart
@@ -70,12 +70,12 @@
return _removePortNameMapping(name);
}
- @FfiNative<Handle Function(Handle)>('IsolateNameServerNatives::LookupPortByName')
+ @Native<Handle Function(Handle)>(symbol: 'IsolateNameServerNatives::LookupPortByName')
external static SendPort? _lookupPortByName(String name);
- @FfiNative<Bool Function(Handle, Handle)>('IsolateNameServerNatives::RegisterPortWithName')
+ @Native<Bool Function(Handle, Handle)>(symbol: 'IsolateNameServerNatives::RegisterPortWithName')
external static bool _registerPortWithName(SendPort port, String name);
- @FfiNative<Bool Function(Handle)>('IsolateNameServerNatives::RemovePortNameMapping')
+ @Native<Bool Function(Handle)>(symbol: 'IsolateNameServerNatives::RemovePortNameMapping')
external static bool _removePortNameMapping(String name);
}
diff --git a/lib/ui/natives.dart b/lib/ui/natives.dart
index 4fb41bf..6a286fa 100644
--- a/lib/ui/natives.dart
+++ b/lib/ui/natives.dart
@@ -18,7 +18,7 @@
_ensureInitialized();
}
}
- @FfiNative<Void Function()>('DartPluginRegistrant_EnsureInitialized')
+ @Native<Void Function()>(symbol: 'DartPluginRegistrant_EnsureInitialized')
external static void _ensureInitialized();
}
@@ -32,10 +32,10 @@
}
class _Logger {
- @FfiNative<Void Function(Handle)>('DartRuntimeHooks::Logger_PrintString')
+ @Native<Void Function(Handle)>(symbol: 'DartRuntimeHooks::Logger_PrintString')
external static void _printString(String? s);
- @FfiNative<Void Function(Handle)>('DartRuntimeHooks::Logger_PrintDebugString')
+ @Native<Void Function(Handle)>(symbol: 'DartRuntimeHooks::Logger_PrintDebugString')
external static void _printDebugString(String? s);
}
@@ -108,13 +108,13 @@
throw UnimplementedError();
}
-@FfiNative<Void Function(Handle)>('DartRuntimeHooks::ScheduleMicrotask')
+@Native<Void Function(Handle)>(symbol: 'DartRuntimeHooks::ScheduleMicrotask')
external void _scheduleMicrotask(void Function() callback);
-@FfiNative<Handle Function(Handle)>('DartRuntimeHooks::GetCallbackHandle')
+@Native<Handle Function(Handle)>(symbol: 'DartRuntimeHooks::GetCallbackHandle')
external int? _getCallbackHandle(Function closure);
-@FfiNative<Handle Function(Int64)>('DartRuntimeHooks::GetCallbackFromHandle')
+@Native<Handle Function(Int64)>(symbol: 'DartRuntimeHooks::GetCallbackFromHandle')
external Function? _getCallbackFromHandle(int handle);
typedef _PrintClosure = void Function(String line);
diff --git a/lib/ui/painting.dart b/lib/ui/painting.dart
index 85ac211..e018f6c 100644
--- a/lib/ui/painting.dart
+++ b/lib/ui/painting.dart
@@ -1879,10 +1879,10 @@
@pragma('vm:entry-point')
_Image._();
- @FfiNative<Int32 Function(Pointer<Void>)>('Image::width', isLeaf: true)
+ @Native<Int32 Function(Pointer<Void>)>(symbol: 'Image::width', isLeaf: true)
external int get width;
- @FfiNative<Int32 Function(Pointer<Void>)>('Image::height', isLeaf: true)
+ @Native<Int32 Function(Pointer<Void>)>(symbol: 'Image::height', isLeaf: true)
external int get height;
Future<ByteData?> toByteData({ImageByteFormat format = ImageByteFormat.rawRgba}) {
@@ -1894,7 +1894,7 @@
}
/// Returns an error message on failure, null on success.
- @FfiNative<Handle Function(Pointer<Void>, Int32, Handle)>('Image::toByteData')
+ @Native<Handle Function(Pointer<Void>, Int32, Handle)>(symbol: 'Image::toByteData')
external String? _toByteData(int format, _Callback<Uint8List?> callback);
bool _disposed = false;
@@ -1913,7 +1913,7 @@
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('Image::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Image::dispose')
external void _dispose();
final Set<Image> _handles = <Image>{};
@@ -2011,7 +2011,7 @@
/// Number of frames in this image.
int get frameCount => _cachedFrameCount ??= _frameCount;
- @FfiNative<Int32 Function(Pointer<Void>)>('Codec::frameCount', isLeaf: true)
+ @Native<Int32 Function(Pointer<Void>)>(symbol: 'Codec::frameCount', isLeaf: true)
external int get _frameCount;
int? _cachedRepetitionCount;
@@ -2021,7 +2021,7 @@
/// * -1 for infinity repetitions.
int get repetitionCount => _cachedRepetitionCount ??= _repetitionCount;
- @FfiNative<Int32 Function(Pointer<Void>)>('Codec::repetitionCount', isLeaf: true)
+ @Native<Int32 Function(Pointer<Void>)>(symbol: 'Codec::repetitionCount', isLeaf: true)
external int get _repetitionCount;
/// Fetches the next animation frame.
@@ -2051,7 +2051,7 @@
}
/// Returns an error message on failure, null on success.
- @FfiNative<Handle Function(Pointer<Void>, Handle)>('Codec::getNextFrame')
+ @Native<Handle Function(Pointer<Void>, Handle)>(symbol: 'Codec::getNextFrame')
external String? _getNextFrame(void Function(_Image?, int) callback);
/// Release the resources used by this object. The object is no longer usable
@@ -2059,7 +2059,7 @@
///
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('Codec::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Codec::dispose')
external void dispose();
}
@@ -2484,7 +2484,7 @@
///
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('EngineLayer::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'EngineLayer::dispose')
external void dispose();
}
@@ -2525,10 +2525,10 @@
return clonedPath;
}
- @FfiNative<Void Function(Handle)>('Path::Create')
+ @Native<Void Function(Handle)>(symbol: 'Path::Create')
external void _constructor();
- @FfiNative<Void Function(Pointer<Void>, Handle)>('Path::clone')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Path::clone')
external void _clone(Path outPath);
/// Determines how the interior of this path is calculated.
@@ -2537,28 +2537,28 @@
PathFillType get fillType => PathFillType.values[_getFillType()];
set fillType(PathFillType value) => _setFillType(value.index);
- @FfiNative<Int32 Function(Pointer<Void>)>('Path::getFillType', isLeaf: true)
+ @Native<Int32 Function(Pointer<Void>)>(symbol: 'Path::getFillType', isLeaf: true)
external int _getFillType();
- @FfiNative<Void Function(Pointer<Void>, Int32)>('Path::setFillType', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Int32)>(symbol: 'Path::setFillType', isLeaf: true)
external void _setFillType(int fillType);
/// Starts a new sub-path at the given coordinate.
- @FfiNative<Void Function(Pointer<Void>, Float, Float)>('Path::moveTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float)>(symbol: 'Path::moveTo', isLeaf: true)
external void moveTo(double x, double y);
/// Starts a new sub-path at the given offset from the current point.
- @FfiNative<Void Function(Pointer<Void>, Float, Float)>('Path::relativeMoveTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float)>(symbol: 'Path::relativeMoveTo', isLeaf: true)
external void relativeMoveTo(double dx, double dy);
/// Adds a straight line segment from the current point to the given
/// point.
- @FfiNative<Void Function(Pointer<Void>, Float, Float)>('Path::lineTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float)>(symbol: 'Path::lineTo', isLeaf: true)
external void lineTo(double x, double y);
/// Adds a straight line segment from the current point to the point
/// at the given offset from the current point.
- @FfiNative<Void Function(Pointer<Void>, Float, Float)>('Path::relativeLineTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float)>(symbol: 'Path::relativeLineTo', isLeaf: true)
external void relativeLineTo(double dx, double dy);
/// Adds a quadratic bezier segment that curves from the current
@@ -2567,14 +2567,14 @@
///
/// 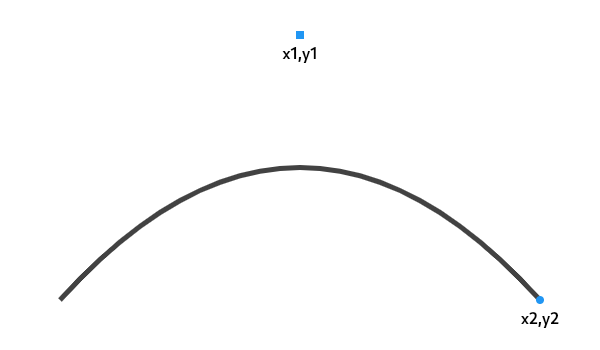
/// 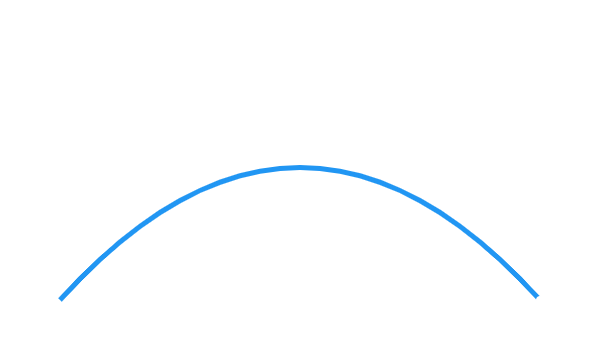
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float)>('Path::quadraticBezierTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float)>(symbol: 'Path::quadraticBezierTo', isLeaf: true)
external void quadraticBezierTo(double x1, double y1, double x2, double y2);
/// Adds a quadratic bezier segment that curves from the current
/// point to the point at the offset (x2,y2) from the current point,
/// using the control point at the offset (x1,y1) from the current
/// point.
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float)>('Path::relativeQuadraticBezierTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float)>(symbol: 'Path::relativeQuadraticBezierTo', isLeaf: true)
external void relativeQuadraticBezierTo(
double x1, double y1, double x2, double y2);
@@ -2584,14 +2584,14 @@
///
/// 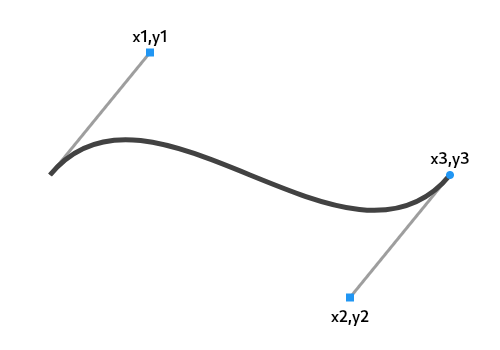
/// 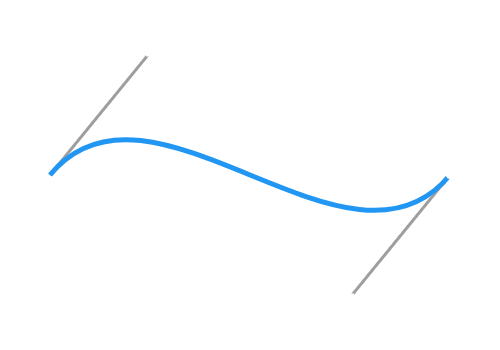
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Float)>('Path::cubicTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Float)>(symbol: 'Path::cubicTo', isLeaf: true)
external void cubicTo(double x1, double y1, double x2, double y2, double x3, double y3);
/// Adds a cubic bezier segment that curves from the current point
/// to the point at the offset (x3,y3) from the current point, using
/// the control points at the offsets (x1,y1) and (x2,y2) from the
/// current point.
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Float)>('Path::relativeCubicTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Float)>(symbol: 'Path::relativeCubicTo', isLeaf: true)
external void relativeCubicTo(double x1, double y1, double x2, double y2, double x3, double y3);
/// Adds a bezier segment that curves from the current point to the
@@ -2602,7 +2602,7 @@
///
/// 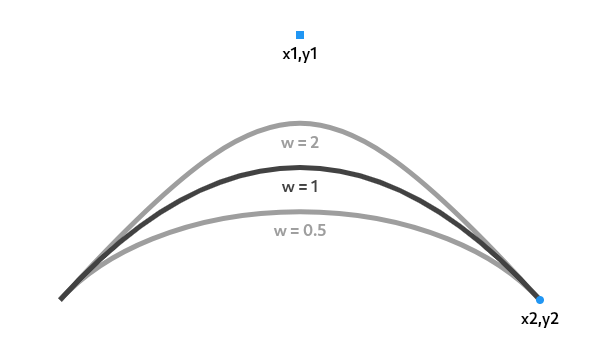
/// 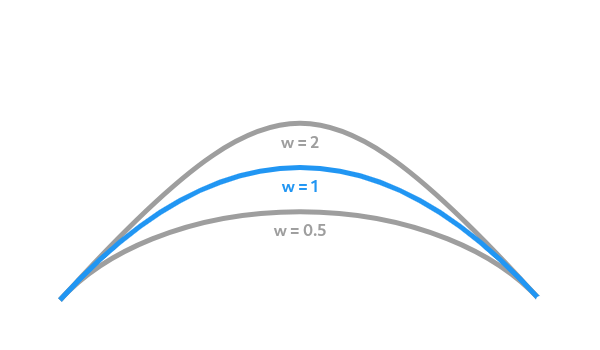
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float, Float)>('Path::conicTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float, Float)>(symbol: 'Path::conicTo', isLeaf: true)
external void conicTo(double x1, double y1, double x2, double y2, double w);
/// Adds a bezier segment that curves from the current point to the
@@ -2611,7 +2611,7 @@
/// the weight w. If the weight is greater than 1, then the curve is
/// a hyperbola; if the weight equals 1, it's a parabola; and if it
/// is less than 1, it is an ellipse.
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float, Float)>('Path::relativeConicTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float, Float)>(symbol: 'Path::relativeConicTo', isLeaf: true)
external void relativeConicTo(double x1, double y1, double x2, double y2, double w);
/// If the `forceMoveTo` argument is false, adds a straight line
@@ -2635,7 +2635,7 @@
_arcTo(rect.left, rect.top, rect.right, rect.bottom, startAngle, sweepAngle, forceMoveTo);
}
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Float, Bool)>('Path::arcTo', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Float, Bool)>(symbol: 'Path::arcTo', isLeaf: true)
external void _arcTo(double left, double top, double right, double bottom, double startAngle, double sweepAngle, bool forceMoveTo);
/// Appends up to four conic curves weighted to describe an oval of `radius`
@@ -2661,7 +2661,7 @@
_arcToPoint(arcEnd.dx, arcEnd.dy, radius.x, radius.y, rotation, largeArc, clockwise);
}
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Bool, Bool)>('Path::arcToPoint', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Bool, Bool)>(symbol: 'Path::arcToPoint', isLeaf: true)
external void _arcToPoint(double arcEndX, double arcEndY, double radiusX, double radiusY, double rotation, bool largeArc, bool clockwise);
/// Appends up to four conic curves weighted to describe an oval of `radius`
@@ -2690,7 +2690,7 @@
_relativeArcToPoint(arcEndDelta.dx, arcEndDelta.dy, radius.x, radius.y, rotation, largeArc, clockwise);
}
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Bool, Bool)>('Path::relativeArcToPoint', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Bool, Bool)>(symbol: 'Path::relativeArcToPoint', isLeaf: true)
external void _relativeArcToPoint(
double arcEndX,
double arcEndY,
@@ -2707,7 +2707,7 @@
_addRect(rect.left, rect.top, rect.right, rect.bottom);
}
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float)>('Path::addRect', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float)>(symbol: 'Path::addRect', isLeaf: true)
external void _addRect(double left, double top, double right, double bottom);
/// Adds a new sub-path that consists of a curve that forms the
@@ -2720,7 +2720,7 @@
_addOval(oval.left, oval.top, oval.right, oval.bottom);
}
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float)>('Path::addOval', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float)>(symbol: 'Path::addOval', isLeaf: true)
external void _addOval(double left, double top, double right, double bottom);
/// Adds a new sub-path with one arc segment that consists of the arc
@@ -2742,7 +2742,7 @@
_addArc(oval.left, oval.top, oval.right, oval.bottom, startAngle, sweepAngle);
}
- @FfiNative<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Float)>('Path::addArc', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Float, Float, Float, Float, Float, Float)>(symbol: 'Path::addArc', isLeaf: true)
external void _addArc(double left, double top, double right, double bottom, double startAngle, double sweepAngle);
/// Adds a new sub-path with a sequence of line segments that connect the given
@@ -2757,7 +2757,7 @@
_addPolygon(_encodePointList(points), close);
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Bool)>('Path::addPolygon')
+ @Native<Void Function(Pointer<Void>, Handle, Bool)>(symbol: 'Path::addPolygon')
external void _addPolygon(Float32List points, bool close);
/// Adds a new sub-path that consists of the straight lines and
@@ -2768,7 +2768,7 @@
_addRRect(rrect._getValue32());
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('Path::addRRect')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Path::addRRect')
external void _addRRect(Float32List rrect);
/// Adds the sub-paths of `path`, offset by `offset`, to this path.
@@ -2787,10 +2787,10 @@
}
}
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Double, Double)>('Path::addPath')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Double, Double)>(symbol: 'Path::addPath')
external void _addPath(Path path, double dx, double dy);
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Double, Double, Handle)>('Path::addPathWithMatrix')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Double, Double, Handle)>(symbol: 'Path::addPathWithMatrix')
external void _addPathWithMatrix(Path path, double dx, double dy, Float64List matrix);
/// Adds the sub-paths of `path`, offset by `offset`, to this path.
@@ -2811,21 +2811,21 @@
}
}
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Double, Double)>('Path::extendWithPath')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Double, Double)>(symbol: 'Path::extendWithPath')
external void _extendWithPath(Path path, double dx, double dy);
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Double, Double, Handle)>('Path::extendWithPathAndMatrix')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Double, Double, Handle)>(symbol: 'Path::extendWithPathAndMatrix')
external void _extendWithPathAndMatrix(Path path, double dx, double dy, Float64List matrix);
/// Closes the last sub-path, as if a straight line had been drawn
/// from the current point to the first point of the sub-path.
- @FfiNative<Void Function(Pointer<Void>)>('Path::close', isLeaf: true)
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Path::close', isLeaf: true)
external void close();
/// Clears the [Path] object of all sub-paths, returning it to the
/// same state it had when it was created. The _current point_ is
/// reset to the origin.
- @FfiNative<Void Function(Pointer<Void>)>('Path::reset', isLeaf: true)
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Path::reset', isLeaf: true)
external void reset();
/// Tests to see if the given point is within the path. (That is, whether the
@@ -2840,7 +2840,7 @@
return _contains(point.dx, point.dy);
}
- @FfiNative<Bool Function(Pointer<Void>, Double, Double)>('Path::contains', isLeaf: true)
+ @Native<Bool Function(Pointer<Void>, Double, Double)>(symbol: 'Path::contains', isLeaf: true)
external bool _contains(double x, double y);
/// Returns a copy of the path with all the segments of every
@@ -2852,7 +2852,7 @@
return path;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Double, Double)>('Path::shift')
+ @Native<Void Function(Pointer<Void>, Handle, Double, Double)>(symbol: 'Path::shift')
external void _shift(Path outPath, double dx, double dy);
/// Returns a copy of the path with all the segments of every
@@ -2864,7 +2864,7 @@
return path;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle)>('Path::transform')
+ @Native<Void Function(Pointer<Void>, Handle, Handle)>(symbol: 'Path::transform')
external void _transform(Path outPath, Float64List matrix4);
/// Computes the bounding rectangle for this path.
@@ -2887,7 +2887,7 @@
return Rect.fromLTRB(rect[0], rect[1], rect[2], rect[3]);
}
- @FfiNative<Handle Function(Pointer<Void>)>('Path::getBounds')
+ @Native<Handle Function(Pointer<Void>)>(symbol: 'Path::getBounds')
external Float32List _getBounds();
/// Combines the two paths according to the manner specified by the given
@@ -2906,7 +2906,7 @@
throw StateError('Path.combine() failed. This may be due an invalid path; in particular, check for NaN values.');
}
- @FfiNative<Bool Function(Pointer<Void>, Pointer<Void>, Pointer<Void>, Int32)>('Path::op')
+ @Native<Bool Function(Pointer<Void>, Pointer<Void>, Pointer<Void>, Int32)>(symbol: 'Path::op')
external bool _op(Path path1, Path path2, int operation);
/// Creates a [PathMetrics] object for this path, which can describe various
@@ -3132,7 +3132,7 @@
_constructor(path, forceClosed);
}
- @FfiNative<Void Function(Handle, Pointer<Void>, Bool)>('PathMeasure::Create')
+ @Native<Void Function(Handle, Pointer<Void>, Bool)>(symbol: 'PathMeasure::Create')
external void _constructor(Path path, bool forceClosed);
double length(int contourIndex) {
@@ -3140,7 +3140,7 @@
return _length(contourIndex);
}
- @FfiNative<Float Function(Pointer<Void>, Int32)>('PathMeasure::getLength', isLeaf: true)
+ @Native<Float Function(Pointer<Void>, Int32)>(symbol: 'PathMeasure::getLength', isLeaf: true)
external double _length(int contourIndex);
Tangent? getTangentForOffset(int contourIndex, double distance) {
@@ -3158,7 +3158,7 @@
}
}
- @FfiNative<Handle Function(Pointer<Void>, Int32, Float)>('PathMeasure::getPosTan')
+ @Native<Handle Function(Pointer<Void>, Int32, Float)>(symbol: 'PathMeasure::getPosTan')
external Float32List _getPosTan(int contourIndex, double distance);
Path extractPath(int contourIndex, double start, double end,
@@ -3169,7 +3169,7 @@
return path;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Int32, Float, Float, Bool)>('PathMeasure::getSegment')
+ @Native<Void Function(Pointer<Void>, Handle, Int32, Float, Float, Bool)>(symbol: 'PathMeasure::getSegment')
external void _extractPath(Path outPath, int contourIndex, double start, double end, bool startWithMoveTo);
bool isClosed(int contourIndex) {
@@ -3177,7 +3177,7 @@
return _isClosed(contourIndex);
}
- @FfiNative<Bool Function(Pointer<Void>, Int32)>('PathMeasure::isClosed', isLeaf: true)
+ @Native<Bool Function(Pointer<Void>, Int32)>(symbol: 'PathMeasure::isClosed', isLeaf: true)
external bool _isClosed(int contourIndex);
// Move to the next contour in the path.
@@ -3192,7 +3192,7 @@
return next;
}
- @FfiNative<Bool Function(Pointer<Void>)>('PathMeasure::nextContour', isLeaf: true)
+ @Native<Bool Function(Pointer<Void>)>(symbol: 'PathMeasure::nextContour', isLeaf: true)
external bool _nativeNextContour();
/// The index of the current contour in the list of contours in the path.
@@ -3502,19 +3502,19 @@
/// the values used for the filter.
final ColorFilter creator;
- @FfiNative<Void Function(Handle)>('ColorFilter::Create')
+ @Native<Void Function(Handle)>(symbol: 'ColorFilter::Create')
external void _constructor();
- @FfiNative<Void Function(Pointer<Void>, Int32, Int32)>('ColorFilter::initMode', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Int32, Int32)>(symbol: 'ColorFilter::initMode', isLeaf: true)
external void _initMode(int color, int blendMode);
- @FfiNative<Void Function(Pointer<Void>, Handle)>('ColorFilter::initMatrix')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'ColorFilter::initMatrix')
external void _initMatrix(Float32List matrix);
- @FfiNative<Void Function(Pointer<Void>)>('ColorFilter::initLinearToSrgbGamma', isLeaf: true)
+ @Native<Void Function(Pointer<Void>)>(symbol: 'ColorFilter::initLinearToSrgbGamma', isLeaf: true)
external void _initLinearToSrgbGamma();
- @FfiNative<Void Function(Pointer<Void>)>('ColorFilter::initSrgbToLinearGamma', isLeaf: true)
+ @Native<Void Function(Pointer<Void>)>(symbol: 'ColorFilter::initSrgbToLinearGamma', isLeaf: true)
external void _initSrgbToLinearGamma();
}
@@ -3815,25 +3815,25 @@
_initComposed(nativeFilterOuter, nativeFilterInner);
}
- @FfiNative<Void Function(Handle)>('ImageFilter::Create')
+ @Native<Void Function(Handle)>(symbol: 'ImageFilter::Create')
external void _constructor();
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Int32)>('ImageFilter::initBlur', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double, Double, Int32)>(symbol: 'ImageFilter::initBlur', isLeaf: true)
external void _initBlur(double sigmaX, double sigmaY, int tileMode);
- @FfiNative<Void Function(Pointer<Void>, Double, Double)>('ImageFilter::initDilate', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'ImageFilter::initDilate', isLeaf: true)
external void _initDilate(double radiusX, double radiusY);
- @FfiNative<Void Function(Pointer<Void>, Double, Double)>('ImageFilter::initErode', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'ImageFilter::initErode', isLeaf: true)
external void _initErode(double radiusX, double radiusY);
- @FfiNative<Void Function(Pointer<Void>, Handle, Int32)>('ImageFilter::initMatrix')
+ @Native<Void Function(Pointer<Void>, Handle, Int32)>(symbol: 'ImageFilter::initMatrix')
external void _initMatrix(Float64List matrix4, int filterQuality);
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>)>('ImageFilter::initColorFilter')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>)>(symbol: 'ImageFilter::initColorFilter')
external void _initColorFilter(_ColorFilter? colorFilter);
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Pointer<Void>)>('ImageFilter::initComposeFilter')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Pointer<Void>)>(symbol: 'ImageFilter::initComposeFilter')
external void _initComposed(_ImageFilter outerFilter, _ImageFilter innerFilter);
/// The original Dart object that created the native wrapper, which retains
@@ -4169,13 +4169,13 @@
_initSweep(center.dx, center.dy, colorsBuffer, colorStopsBuffer, tileMode.index, startAngle, endAngle, matrix4);
}
- @FfiNative<Void Function(Handle)>('Gradient::Create')
+ @Native<Void Function(Handle)>(symbol: 'Gradient::Create')
external void _constructor();
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle, Handle, Int32, Handle)>('Gradient::initLinear')
+ @Native<Void Function(Pointer<Void>, Handle, Handle, Handle, Int32, Handle)>(symbol: 'Gradient::initLinear')
external void _initLinear(Float32List endPoints, Int32List colors, Float32List? colorStops, int tileMode, Float64List? matrix4);
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Handle, Handle, Int32, Handle)>('Gradient::initRadial')
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Handle, Handle, Int32, Handle)>(symbol: 'Gradient::initRadial')
external void _initRadial(
double centerX,
double centerY,
@@ -4185,8 +4185,7 @@
int tileMode,
Float64List? matrix4);
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double, Handle, Handle, Int32, Handle)>(
- 'Gradient::initTwoPointConical')
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double, Handle, Handle, Int32, Handle)>(symbol: 'Gradient::initTwoPointConical')
external void _initConical(
double startX,
double startY,
@@ -4199,7 +4198,7 @@
int tileMode,
Float64List? matrix4);
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Handle, Handle, Int32, Double, Double, Handle)>('Gradient::initSweep')
+ @Native<Void Function(Pointer<Void>, Double, Double, Handle, Handle, Int32, Double, Double, Handle)>(symbol: 'Gradient::initSweep')
external void _initSweep(
double centerX,
double centerY,
@@ -4271,15 +4270,15 @@
_dispose();
}
- @FfiNative<Void Function(Handle)>('ImageShader::Create')
+ @Native<Void Function(Handle)>(symbol: 'ImageShader::Create')
external void _constructor();
- @FfiNative<Handle Function(Pointer<Void>, Pointer<Void>, Int32, Int32, Int32, Handle)>('ImageShader::initWithImage')
+ @Native<Handle Function(Pointer<Void>, Pointer<Void>, Int32, Int32, Int32, Handle)>(symbol: 'ImageShader::initWithImage')
external String? _initWithImage(_Image image, int tmx, int tmy, int filterQualityIndex, Float64List matrix4);
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('ImageShader::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'ImageShader::dispose')
external void _dispose();
}
@@ -4362,10 +4361,10 @@
@pragma('vm:entry-point')
late int _samplerCount;
- @FfiNative<Void Function(Handle)>('FragmentProgram::Create')
+ @Native<Void Function(Handle)>(symbol: 'FragmentProgram::Create')
external void _constructor();
- @FfiNative<Handle Function(Pointer<Void>, Handle)>('FragmentProgram::initFromAsset')
+ @Native<Handle Function(Pointer<Void>, Handle)>(symbol: 'FragmentProgram::initFromAsset')
external String _initFromAsset(String assetKey);
/// Returns a fresh instance of [FragmentShader].
@@ -4471,16 +4470,16 @@
_dispose();
}
- @FfiNative<Handle Function(Handle, Handle, Handle, Handle)>('ReusableFragmentShader::Create')
+ @Native<Handle Function(Handle, Handle, Handle, Handle)>(symbol: 'ReusableFragmentShader::Create')
external Float32List _constructor(FragmentProgram program, int floatUniforms, int samplerUniforms);
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle)>('ReusableFragmentShader::SetImageSampler')
+ @Native<Void Function(Pointer<Void>, Handle, Handle)>(symbol: 'ReusableFragmentShader::SetImageSampler')
external void _setImageSampler(int index, _Image sampler);
- @FfiNative<Bool Function(Pointer<Void>)>('ReusableFragmentShader::ValidateSamplers')
+ @Native<Bool Function(Pointer<Void>)>(symbol: 'ReusableFragmentShader::ValidateSamplers')
external bool _validateSamplers();
- @FfiNative<Void Function(Pointer<Void>)>('ReusableFragmentShader::Dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'ReusableFragmentShader::Dispose')
external void _dispose();
}
@@ -4701,7 +4700,7 @@
}
}
- @FfiNative<Bool Function(Handle, Int32, Handle, Handle, Handle, Handle)>('Vertices::init')
+ @Native<Bool Function(Handle, Int32, Handle, Handle, Handle, Handle)>(symbol: 'Vertices::init')
external static bool _init(Vertices outVertices,
int mode,
Float32List positions,
@@ -4722,7 +4721,7 @@
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('Vertices::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Vertices::dispose')
external void _dispose();
bool _disposed = false;
@@ -4824,7 +4823,7 @@
_constructor(recorder, cullRect.left, cullRect.top, cullRect.right, cullRect.bottom);
}
- @FfiNative<Void Function(Handle, Pointer<Void>, Double, Double, Double, Double)>('Canvas::Create')
+ @Native<Void Function(Handle, Pointer<Void>, Double, Double, Double, Double)>(symbol: 'Canvas::Create')
external void _constructor(PictureRecorder recorder, double left, double top, double right, double bottom);
// The underlying Skia SkCanvas is owned by the PictureRecorder used to create this Canvas.
@@ -4840,7 +4839,7 @@
///
/// * [saveLayer], which does the same thing but additionally also groups the
/// commands done until the matching [restore].
- @FfiNative<Void Function(Pointer<Void>)>('Canvas::save', isLeaf: true)
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Canvas::save', isLeaf: true)
external void save();
/// Saves a copy of the current transform and clip on the save stack, and then
@@ -4962,10 +4961,10 @@
}
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle)>('Canvas::saveLayerWithoutBounds')
+ @Native<Void Function(Pointer<Void>, Handle, Handle)>(symbol: 'Canvas::saveLayerWithoutBounds')
external void _saveLayerWithoutBounds(List<Object?>? paintObjects, ByteData paintData);
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>('Canvas::saveLayer')
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::saveLayer')
external void _saveLayer(double left, double top, double right, double bottom, List<Object?>? paintObjects, ByteData paintData);
/// Pops the current save stack, if there is anything to pop.
@@ -4975,7 +4974,7 @@
///
/// If the state was pushed with with [saveLayer], then this call will also
/// cause the new layer to be composited into the previous layer.
- @FfiNative<Void Function(Pointer<Void>)>('Canvas::restore', isLeaf: true)
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Canvas::restore', isLeaf: true)
external void restore();
/// Restores the save stack to a previous level as might be obtained from [getSaveCount].
@@ -4987,7 +4986,7 @@
/// If any of the state stack levels restored by this call were pushed with
/// [saveLayer], then this call will also cause those layers to be composited
/// into their previous layers.
- @FfiNative<Void Function(Pointer<Void>, Int32)>('Canvas::restoreToCount', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Int32)>(symbol: 'Canvas::restoreToCount', isLeaf: true)
external void restoreToCount(int count);
/// Returns the number of items on the save stack, including the
@@ -4996,12 +4995,12 @@
/// each matching call to [restore] decrements it.
///
/// This number cannot go below 1.
- @FfiNative<Int32 Function(Pointer<Void>)>('Canvas::getSaveCount', isLeaf: true)
+ @Native<Int32 Function(Pointer<Void>)>(symbol: 'Canvas::getSaveCount', isLeaf: true)
external int getSaveCount();
/// Add a translation to the current transform, shifting the coordinate space
/// horizontally by the first argument and vertically by the second argument.
- @FfiNative<Void Function(Pointer<Void>, Double, Double)>('Canvas::translate', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Canvas::translate', isLeaf: true)
external void translate(double dx, double dy);
/// Add an axis-aligned scale to the current transform, scaling by the first
@@ -5012,18 +5011,18 @@
/// directions.
void scale(double sx, [double? sy]) => _scale(sx, sy ?? sx);
- @FfiNative<Void Function(Pointer<Void>, Double, Double)>('Canvas::scale', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Canvas::scale', isLeaf: true)
external void _scale(double sx, double sy);
/// Add a rotation to the current transform. The argument is in radians clockwise.
- @FfiNative<Void Function(Pointer<Void>, Double)>('Canvas::rotate', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double)>(symbol: 'Canvas::rotate', isLeaf: true)
external void rotate(double radians);
/// Add an axis-aligned skew to the current transform, with the first argument
/// being the horizontal skew in rise over run units clockwise around the
/// origin, and the second argument being the vertical skew in rise over run
/// units clockwise around the origin.
- @FfiNative<Void Function(Pointer<Void>, Double, Double)>('Canvas::skew', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Canvas::skew', isLeaf: true)
external void skew(double sx, double sy);
/// Multiply the current transform by the specified 4⨉4 transformation matrix
@@ -5036,7 +5035,7 @@
_transform(matrix4);
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('Canvas::transform')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Canvas::transform')
external void _transform(Float64List matrix4);
/// Returns the current transform including the combined result of all transform
@@ -5053,7 +5052,7 @@
return matrix4;
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('Canvas::getTransform')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Canvas::getTransform')
external void _getTransform(Float64List matrix4);
/// Reduces the clip region to the intersection of the current clip and the
@@ -5076,7 +5075,7 @@
_clipRect(rect.left, rect.top, rect.right, rect.bottom, clipOp.index, doAntiAlias);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Double, Int32, Bool)>('Canvas::clipRect', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Int32, Bool)>(symbol: 'Canvas::clipRect', isLeaf: true)
external void _clipRect(double left, double top, double right, double bottom, int clipOp, bool doAntiAlias);
/// Reduces the clip region to the intersection of the current clip and the
@@ -5095,7 +5094,7 @@
_clipRRect(rrect._getValue32(), doAntiAlias);
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Bool)>('Canvas::clipRRect')
+ @Native<Void Function(Pointer<Void>, Handle, Bool)>(symbol: 'Canvas::clipRRect')
external void _clipRRect(Float32List rrect, bool doAntiAlias);
/// Reduces the clip region to the intersection of the current clip and the
@@ -5114,7 +5113,7 @@
_clipPath(path, doAntiAlias);
}
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Bool)>('Canvas::clipPath')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Bool)>(symbol: 'Canvas::clipPath')
external void _clipPath(Path path, bool doAntiAlias);
/// Returns the conservative bounds of the combined result of all clip methods
@@ -5175,7 +5174,7 @@
return Rect.fromLTRB(bounds[0], bounds[1], bounds[2], bounds[3]);
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('Canvas::getLocalClipBounds')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Canvas::getLocalClipBounds')
external void _getLocalClipBounds(Float64List bounds);
/// Returns the conservative bounds of the combined result of all clip methods
@@ -5198,7 +5197,7 @@
return Rect.fromLTRB(bounds[0], bounds[1], bounds[2], bounds[3]);
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('Canvas::getDestinationClipBounds')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Canvas::getDestinationClipBounds')
external void _getDestinationClipBounds(Float64List bounds);
/// Paints the given [Color] onto the canvas, applying the given
@@ -5210,7 +5209,7 @@
_drawColor(color.value, blendMode.index);
}
- @FfiNative<Void Function(Pointer<Void>, Uint32, Int32)>('Canvas::drawColor', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Uint32, Int32)>(symbol: 'Canvas::drawColor', isLeaf: true)
external void _drawColor(int color, int blendMode);
/// Draws a line between the given points using the given paint. The line is
@@ -5227,7 +5226,7 @@
_drawLine(p1.dx, p1.dy, p2.dx, p2.dy, paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>('Canvas::drawLine')
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::drawLine')
external void _drawLine(double x1, double y1, double x2, double y2, List<Object?>? paintObjects, ByteData paintData);
/// Fills the canvas with the given [Paint].
@@ -5239,7 +5238,7 @@
_drawPaint(paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle)>('Canvas::drawPaint')
+ @Native<Void Function(Pointer<Void>, Handle, Handle)>(symbol: 'Canvas::drawPaint')
external void _drawPaint(List<Object?>? paintObjects, ByteData paintData);
/// Draws a rectangle with the given [Paint]. Whether the rectangle is filled
@@ -5253,7 +5252,7 @@
_drawRect(rect.left, rect.top, rect.right, rect.bottom, paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>('Canvas::drawRect')
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::drawRect')
external void _drawRect(double left, double top, double right, double bottom, List<Object?>? paintObjects, ByteData paintData);
/// Draws a rounded rectangle with the given [Paint]. Whether the rectangle is
@@ -5267,7 +5266,7 @@
_drawRRect(rrect._getValue32(), paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle, Handle)>('Canvas::drawRRect')
+ @Native<Void Function(Pointer<Void>, Handle, Handle, Handle)>(symbol: 'Canvas::drawRRect')
external void _drawRRect(Float32List rrect, List<Object?>? paintObjects, ByteData paintData);
/// Draws a shape consisting of the difference between two rounded rectangles
@@ -5282,7 +5281,7 @@
_drawDRRect(outer._getValue32(), inner._getValue32(), paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle, Handle, Handle)>('Canvas::drawDRRect')
+ @Native<Void Function(Pointer<Void>, Handle, Handle, Handle, Handle)>(symbol: 'Canvas::drawDRRect')
external void _drawDRRect(Float32List outer, Float32List inner, List<Object?>? paintObjects, ByteData paintData);
/// Draws an axis-aligned oval that fills the given axis-aligned rectangle
@@ -5297,7 +5296,7 @@
_drawOval(rect.left, rect.top, rect.right, rect.bottom, paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>('Canvas::drawOval')
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::drawOval')
external void _drawOval(double left, double top, double right, double bottom, List<Object?>? paintObjects, ByteData paintData);
/// Draws a circle centered at the point given by the first argument and
@@ -5313,7 +5312,7 @@
_drawCircle(c.dx, c.dy, radius, paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Handle, Handle)>('Canvas::drawCircle')
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::drawCircle')
external void _drawCircle(double x, double y, double radius, List<Object?>? paintObjects, ByteData paintData);
/// Draw an arc scaled to fit inside the given rectangle.
@@ -5336,7 +5335,7 @@
_drawArc(rect.left, rect.top, rect.right, rect.bottom, startAngle, sweepAngle, useCenter, paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double, Bool, Handle, Handle)>('Canvas::drawArc')
+ @Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double, Bool, Handle, Handle)>(symbol: 'Canvas::drawArc')
external void _drawArc(
double left,
double top,
@@ -5359,7 +5358,7 @@
_drawPath(path, paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Handle, Handle)>('Canvas::drawPath')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Handle, Handle)>(symbol: 'Canvas::drawPath')
external void _drawPath(Path path, List<Object?>? paintObjects, ByteData paintData);
/// Draws the given [Image] into the canvas with its top-left corner at the
@@ -5375,7 +5374,7 @@
}
}
- @FfiNative<Handle Function(Pointer<Void>, Pointer<Void>, Double, Double, Handle, Handle, Int32)>('Canvas::drawImage')
+ @Native<Handle Function(Pointer<Void>, Pointer<Void>, Double, Double, Handle, Handle, Int32)>(symbol: 'Canvas::drawImage')
external String? _drawImage(_Image image, double x, double y, List<Object?>? paintObjects, ByteData paintData, int filterQualityIndex);
/// Draws the subset of the given image described by the `src` argument into
@@ -5410,7 +5409,7 @@
}
}
- @FfiNative<Handle Function(Pointer<Void>, Pointer<Void>, Double, Double, Double, Double, Double, Double, Double, Double, Handle, Handle, Int32)>('Canvas::drawImageRect')
+ @Native<Handle Function(Pointer<Void>, Pointer<Void>, Double, Double, Double, Double, Double, Double, Double, Double, Handle, Handle, Int32)>(symbol: 'Canvas::drawImageRect')
external String? _drawImageRect(
_Image image,
double srcLeft,
@@ -5461,7 +5460,7 @@
}
}
- @FfiNative<Handle Function(Pointer<Void>, Pointer<Void>, Double, Double, Double, Double, Double, Double, Double, Double, Handle, Handle, Int32)>('Canvas::drawImageNine')
+ @Native<Handle Function(Pointer<Void>, Pointer<Void>, Double, Double, Double, Double, Double, Double, Double, Double, Handle, Handle, Int32)>(symbol: 'Canvas::drawImageNine')
external String? _drawImageNine(
_Image image,
double centerLeft,
@@ -5484,7 +5483,7 @@
_drawPicture(picture);
}
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>)>('Canvas::drawPicture')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>)>(symbol: 'Canvas::drawPicture')
external void _drawPicture(Picture picture);
/// Draws the text in the given [Paragraph] into this canvas at the given
@@ -5555,7 +5554,7 @@
_drawPoints(paint._objects, paint._data, pointMode.index, points);
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Handle, Int32, Handle)>('Canvas::drawPoints')
+ @Native<Void Function(Pointer<Void>, Handle, Handle, Int32, Handle)>(symbol: 'Canvas::drawPoints')
external void _drawPoints(List<Object?>? paintObjects, ByteData paintData, int pointMode, Float32List points);
/// Draws a set of [Vertices] onto the canvas as one or more triangles.
@@ -5594,7 +5593,7 @@
_drawVertices(vertices, blendMode.index, paint._objects, paint._data);
}
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Int32, Handle, Handle)>('Canvas::drawVertices')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Int32, Handle, Handle)>(symbol: 'Canvas::drawVertices')
external void _drawVertices(Vertices vertices, int blendMode, List<Object?>? paintObjects, ByteData paintData);
/// Draws many parts of an image - the [atlas] - onto the canvas.
@@ -5967,7 +5966,7 @@
}
}
- @FfiNative<Handle Function(Pointer<Void>, Handle, Handle, Int32, Pointer<Void>, Handle, Handle, Handle, Int32, Handle)>('Canvas::drawAtlas')
+ @Native<Handle Function(Pointer<Void>, Handle, Handle, Int32, Pointer<Void>, Handle, Handle, Handle, Int32, Handle)>(symbol: 'Canvas::drawAtlas')
external String? _drawAtlas(
List<Object?>? paintObjects,
ByteData paintData,
@@ -5992,7 +5991,7 @@
_drawShadow(path, color.value, elevation, transparentOccluder);
}
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Uint32, Double, Bool)>('Canvas::drawShadow')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Uint32, Double, Bool)>(symbol: 'Canvas::drawShadow')
external void _drawShadow(Path path, int color, double elevation, bool transparentOccluder);
}
@@ -6050,7 +6049,7 @@
);
}
- @FfiNative<Handle Function(Pointer<Void>, Uint32, Uint32, Handle)>('Picture::toImage')
+ @Native<Handle Function(Pointer<Void>, Uint32, Uint32, Handle)>(symbol: 'Picture::toImage')
external String? _toImage(int width, int height, void Function(_Image?) callback);
/// Synchronously creates a handle to an image of this picture.
@@ -6081,7 +6080,7 @@
return Image._(image, image.width, image.height);
}
- @FfiNative<Void Function(Pointer<Void>, Uint32, Uint32, Handle)>('Picture::toImageSync')
+ @Native<Void Function(Pointer<Void>, Uint32, Uint32, Handle)>(symbol: 'Picture::toImageSync')
external void _toImageSync(int width, int height, _Image outImage);
/// Release the resources used by this object. The object is no longer usable
@@ -6098,7 +6097,7 @@
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('Picture::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Picture::dispose')
external void _dispose();
bool _disposed = false;
@@ -6119,7 +6118,7 @@
///
/// The actual size of this picture may be larger, particularly if it contains
/// references to image or other large objects.
- @FfiNative<Uint64 Function(Pointer<Void>)>('Picture::GetAllocationSize', isLeaf: true)
+ @Native<Uint64 Function(Pointer<Void>)>(symbol: 'Picture::GetAllocationSize', isLeaf: true)
external int get approximateBytesUsed;
}
@@ -6134,7 +6133,7 @@
@pragma('vm:entry-point')
PictureRecorder() { _constructor(); }
- @FfiNative<Void Function(Handle)>('PictureRecorder::Create')
+ @Native<Void Function(Handle)>(symbol: 'PictureRecorder::Create')
external void _constructor();
/// Whether this object is currently recording commands.
@@ -6165,7 +6164,7 @@
return picture;
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('PictureRecorder::endRecording')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'PictureRecorder::endRecording')
external void _endRecording(Picture outPicture);
Canvas? _canvas;
@@ -6426,13 +6425,13 @@
});
}
- @FfiNative<Handle Function(Handle, Handle, Handle)>('ImmutableBuffer::init')
+ @Native<Handle Function(Handle, Handle, Handle)>(symbol: 'ImmutableBuffer::init')
external String? _init(Uint8List list, _Callback<void> callback);
- @FfiNative<Handle Function(Handle, Handle, Handle)>('ImmutableBuffer::initFromAsset')
+ @Native<Handle Function(Handle, Handle, Handle)>(symbol: 'ImmutableBuffer::initFromAsset')
external String? _initFromAsset(String assetKey, _Callback<int> callback);
- @FfiNative<Handle Function(Handle, Handle, Handle)>('ImmutableBuffer::initFromFile')
+ @Native<Handle Function(Handle, Handle, Handle)>(symbol: 'ImmutableBuffer::initFromFile')
external String? _initFromFile(String assetKey, _Callback<int> callback);
/// The length, in bytes, of the underlying data.
@@ -6473,7 +6472,7 @@
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('ImmutableBuffer::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'ImmutableBuffer::dispose')
external void _dispose();
}
@@ -6516,15 +6515,15 @@
}).then((_) => descriptor);
}
- @FfiNative<Handle Function(Handle, Pointer<Void>, Handle)>('ImageDescriptor::initEncoded')
+ @Native<Handle Function(Handle, Pointer<Void>, Handle)>(symbol: 'ImageDescriptor::initEncoded')
external String? _initEncoded(ImmutableBuffer buffer, _Callback<void> callback);
- @FfiNative<Void Function(Handle, Handle, Int32, Int32, Int32, Int32)>('ImageDescriptor::initRaw')
+ @Native<Void Function(Handle, Handle, Int32, Int32, Int32, Int32)>(symbol: 'ImageDescriptor::initRaw')
external static void _initRaw(ImageDescriptor outDescriptor, ImmutableBuffer buffer, int width, int height, int rowBytes, int pixelFormat);
int? _width;
- @FfiNative<Int32 Function(Pointer<Void>)>('ImageDescriptor::width', isLeaf: true)
+ @Native<Int32 Function(Pointer<Void>)>(symbol: 'ImageDescriptor::width', isLeaf: true)
external int _getWidth();
/// The width, in pixels, of the image.
@@ -6534,7 +6533,7 @@
int? _height;
- @FfiNative<Int32 Function(Pointer<Void>)>('ImageDescriptor::height', isLeaf: true)
+ @Native<Int32 Function(Pointer<Void>)>(symbol: 'ImageDescriptor::height', isLeaf: true)
external int _getHeight();
/// The height, in pixels, of the image.
@@ -6544,7 +6543,7 @@
int? _bytesPerPixel;
- @FfiNative<Int32 Function(Pointer<Void>)>('ImageDescriptor::bytesPerPixel', isLeaf: true)
+ @Native<Int32 Function(Pointer<Void>)>(symbol: 'ImageDescriptor::bytesPerPixel', isLeaf: true)
external int _getBytesPerPixel();
/// The number of bytes per pixel in the image.
@@ -6557,7 +6556,7 @@
///
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('ImageDescriptor::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'ImageDescriptor::dispose')
external void dispose();
/// Creates a [Codec] object which is suitable for decoding the data in the
@@ -6595,7 +6594,7 @@
return codec;
}
- @FfiNative<Void Function(Pointer<Void>, Handle, Int32, Int32)>('ImageDescriptor::instantiateCodec')
+ @Native<Void Function(Pointer<Void>, Handle, Int32, Int32)>(symbol: 'ImageDescriptor::instantiateCodec')
external void _instantiateCodec(Codec outCodec, int targetWidth, int targetHeight);
}
diff --git a/lib/ui/platform_dispatcher.dart b/lib/ui/platform_dispatcher.dart
index b3b4de7..9cce6b3 100644
--- a/lib/ui/platform_dispatcher.dart
+++ b/lib/ui/platform_dispatcher.dart
@@ -87,7 +87,7 @@
return token == 0 ? null : RootIsolateToken._(token);
}();
- @FfiNative<Int64 Function()>('PlatformConfigurationNativeApi::GetRootIsolateToken')
+ @Native<Int64 Function()>(symbol: 'PlatformConfigurationNativeApi::GetRootIsolateToken')
external static int __getRootIsolateToken();
}
@@ -520,7 +520,7 @@
void _nativeSetNeedsReportTimings(bool value) => __nativeSetNeedsReportTimings(value);
- @FfiNative<Void Function(Bool)>('PlatformConfigurationNativeApi::SetNeedsReportTimings')
+ @Native<Void Function(Bool)>(symbol: 'PlatformConfigurationNativeApi::SetNeedsReportTimings')
external static void __nativeSetNeedsReportTimings(bool value);
// Called from the engine, via hooks.dart
@@ -553,7 +553,7 @@
String? _sendPlatformMessage(String name, PlatformMessageResponseCallback? callback, ByteData? data) =>
__sendPlatformMessage(name, callback, data);
- @FfiNative<Handle Function(Handle, Handle, Handle)>('PlatformConfigurationNativeApi::SendPlatformMessage')
+ @Native<Handle Function(Handle, Handle, Handle)>(symbol: 'PlatformConfigurationNativeApi::SendPlatformMessage')
external static String? __sendPlatformMessage(String name, PlatformMessageResponseCallback? callback, ByteData? data);
/// Sends a message to a platform-specific plugin via a [SendPort].
@@ -579,7 +579,7 @@
String? _sendPortPlatformMessage(String name, int identifier, int port, ByteData? data) =>
__sendPortPlatformMessage(name, identifier, port, data);
- @FfiNative<Handle Function(Handle, Handle, Handle, Handle)>('PlatformConfigurationNativeApi::SendPortPlatformMessage')
+ @Native<Handle Function(Handle, Handle, Handle, Handle)>(symbol: 'PlatformConfigurationNativeApi::SendPortPlatformMessage')
external static String? __sendPortPlatformMessage(String name, int identifier, int port, ByteData? data);
/// Registers the current isolate with the isolate identified with by the
@@ -589,7 +589,7 @@
DartPluginRegistrant.ensureInitialized();
__registerBackgroundIsolate(token._token);
}
- @FfiNative<Void Function(Int64)>('PlatformConfigurationNativeApi::RegisterBackgroundIsolate')
+ @Native<Void Function(Int64)>(symbol: 'PlatformConfigurationNativeApi::RegisterBackgroundIsolate')
external static void __registerBackgroundIsolate(int rootIsolateId);
/// Called whenever this platform dispatcher receives a message from a
@@ -618,7 +618,7 @@
/// Called by [_dispatchPlatformMessage].
void _respondToPlatformMessage(int responseId, ByteData? data) => __respondToPlatformMessage(responseId, data);
- @FfiNative<Void Function(IntPtr, Handle)>('PlatformConfigurationNativeApi::RespondToPlatformMessage')
+ @Native<Void Function(IntPtr, Handle)>(symbol: 'PlatformConfigurationNativeApi::RespondToPlatformMessage')
external static void __respondToPlatformMessage(int responseId, ByteData? data);
/// Wraps the given [callback] in another callback that ensures that the
@@ -678,7 +678,7 @@
/// Note that this does not rename any child isolates of the root.
void setIsolateDebugName(String name) => _setIsolateDebugName(name);
- @FfiNative<Void Function(Handle)>('PlatformConfigurationNativeApi::SetIsolateDebugName')
+ @Native<Void Function(Handle)>(symbol: 'PlatformConfigurationNativeApi::SetIsolateDebugName')
external static void _setIsolateDebugName(String name);
/// Requests the Dart VM to adjusts the GC heuristics based on the requested `performance_mode`.
@@ -691,7 +691,7 @@
_requestDartPerformanceMode(mode.index);
}
- @FfiNative<Int Function(Int)>('PlatformConfigurationNativeApi::RequestDartPerformanceMode')
+ @Native<Int Function(Int)>(symbol: 'PlatformConfigurationNativeApi::RequestDartPerformanceMode')
external static int _requestDartPerformanceMode(int mode);
/// The embedder can specify data that the isolate can request synchronously
@@ -705,7 +705,7 @@
/// platform channel may be used.
ByteData? getPersistentIsolateData() => _getPersistentIsolateData();
- @FfiNative<Handle Function()>('PlatformConfigurationNativeApi::GetPersistentIsolateData')
+ @Native<Handle Function()>(symbol: 'PlatformConfigurationNativeApi::GetPersistentIsolateData')
external static ByteData? _getPersistentIsolateData();
/// Requests that, at the next appropriate opportunity, the [onBeginFrame] and
@@ -717,7 +717,7 @@
/// scheduling of frames.
void scheduleFrame() => _scheduleFrame();
- @FfiNative<Void Function()>('PlatformConfigurationNativeApi::ScheduleFrame')
+ @Native<Void Function()>(symbol: 'PlatformConfigurationNativeApi::ScheduleFrame')
external static void _scheduleFrame();
/// Additional accessibility features that may be enabled by the platform.
@@ -768,7 +768,7 @@
''')
void updateSemantics(SemanticsUpdate update) => _updateSemantics(update);
- @FfiNative<Void Function(Pointer<Void>)>('PlatformConfigurationNativeApi::UpdateSemantics')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'PlatformConfigurationNativeApi::UpdateSemantics')
external static void _updateSemantics(SemanticsUpdate update);
/// The system-reported default locale of the device.
@@ -830,7 +830,7 @@
List<String> _computePlatformResolvedLocale(List<String?> supportedLocalesData) => __computePlatformResolvedLocale(supportedLocalesData);
- @FfiNative<Handle Function(Handle)>('PlatformConfigurationNativeApi::ComputePlatformResolvedLocale')
+ @Native<Handle Function(Handle)>(symbol: 'PlatformConfigurationNativeApi::ComputePlatformResolvedLocale')
external static List<String> __computePlatformResolvedLocale(List<String?> supportedLocalesData);
/// A callback that is invoked whenever [locale] changes value.
@@ -1213,7 +1213,7 @@
/// requests from the embedder.
String get defaultRouteName => _defaultRouteName();
- @FfiNative<Handle Function()>('PlatformConfigurationNativeApi::DefaultRouteName')
+ @Native<Handle Function()>(symbol: 'PlatformConfigurationNativeApi::DefaultRouteName')
external static String _defaultRouteName();
}
diff --git a/lib/ui/semantics.dart b/lib/ui/semantics.dart
index 9bf8d2b..feb9b82 100644
--- a/lib/ui/semantics.dart
+++ b/lib/ui/semantics.dart
@@ -712,7 +712,7 @@
_initSpellOutStringAttribute(this, range.start, range.end);
}
- @FfiNative<Void Function(Handle, Int32, Int32)>('NativeStringAttribute::initSpellOutStringAttribute')
+ @Native<Void Function(Handle, Int32, Int32)>(symbol: 'NativeStringAttribute::initSpellOutStringAttribute')
external static void _initSpellOutStringAttribute(SpellOutStringAttribute instance, int start, int end);
@override
@@ -748,7 +748,7 @@
/// The lanuage of this attribute.
final Locale locale;
- @FfiNative<Void Function(Handle, Int32, Int32, Handle)>('NativeStringAttribute::initLocaleStringAttribute')
+ @Native<Void Function(Handle, Int32, Int32, Handle)>(symbol: 'NativeStringAttribute::initLocaleStringAttribute')
external static void _initLocaleStringAttribute(LocaleStringAttribute instance, int start, int end, String locale);
@override
@@ -773,7 +773,7 @@
@pragma('vm:entry-point')
SemanticsUpdateBuilder() { _constructor(); }
- @FfiNative<Void Function(Handle)>('SemanticsUpdateBuilder::Create')
+ @Native<Void Function(Handle)>(symbol: 'SemanticsUpdateBuilder::Create')
external void _constructor();
/// Update the information associated with the node with the given `id`.
@@ -934,7 +934,7 @@
additionalActions,
);
}
- @FfiNative<
+ @Native<
Void Function(
Pointer<Void>,
Int32,
@@ -971,7 +971,7 @@
Handle,
Handle,
Handle,
- Handle)>('SemanticsUpdateBuilder::updateNode')
+ Handle)>(symbol: 'SemanticsUpdateBuilder::updateNode')
external void _updateNode(
int id,
int flags,
@@ -1029,7 +1029,7 @@
assert(overrideId != null);
_updateCustomAction(id, label ?? '', hint ?? '', overrideId);
}
- @FfiNative<Void Function(Pointer<Void>, Int32, Handle, Handle, Int32)>('SemanticsUpdateBuilder::updateCustomAction')
+ @Native<Void Function(Pointer<Void>, Int32, Handle, Handle, Int32)>(symbol: 'SemanticsUpdateBuilder::updateCustomAction')
external void _updateCustomAction(int id, String label, String hint, int overrideId);
/// Creates a [SemanticsUpdate] object that encapsulates the updates recorded
@@ -1044,7 +1044,7 @@
_build(semanticsUpdate);
return semanticsUpdate;
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('SemanticsUpdateBuilder::build')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'SemanticsUpdateBuilder::build')
external void _build(SemanticsUpdate outSemanticsUpdate);
}
@@ -1070,6 +1070,6 @@
///
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('SemanticsUpdate::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'SemanticsUpdate::dispose')
external void dispose();
}
diff --git a/lib/ui/text.dart b/lib/ui/text.dart
index 69983ae..2a0c4c3 100644
--- a/lib/ui/text.dart
+++ b/lib/ui/text.dart
@@ -2697,44 +2697,44 @@
/// The amount of horizontal space this paragraph occupies.
///
/// Valid only after [layout] has been called.
- @FfiNative<Double Function(Pointer<Void>)>('Paragraph::width', isLeaf: true)
+ @Native<Double Function(Pointer<Void>)>(symbol: 'Paragraph::width', isLeaf: true)
external double get width;
/// The amount of vertical space this paragraph occupies.
///
/// Valid only after [layout] has been called.
- @FfiNative<Double Function(Pointer<Void>)>('Paragraph::height', isLeaf: true)
+ @Native<Double Function(Pointer<Void>)>(symbol: 'Paragraph::height', isLeaf: true)
external double get height;
/// The distance from the left edge of the leftmost glyph to the right edge of
/// the rightmost glyph in the paragraph.
///
/// Valid only after [layout] has been called.
- @FfiNative<Double Function(Pointer<Void>)>('Paragraph::longestLine', isLeaf: true)
+ @Native<Double Function(Pointer<Void>)>(symbol: 'Paragraph::longestLine', isLeaf: true)
external double get longestLine;
/// The minimum width that this paragraph could be without failing to paint
/// its contents within itself.
///
/// Valid only after [layout] has been called.
- @FfiNative<Double Function(Pointer<Void>)>('Paragraph::minIntrinsicWidth', isLeaf: true)
+ @Native<Double Function(Pointer<Void>)>(symbol: 'Paragraph::minIntrinsicWidth', isLeaf: true)
external double get minIntrinsicWidth;
/// Returns the smallest width beyond which increasing the width never
/// decreases the height.
///
/// Valid only after [layout] has been called.
- @FfiNative<Double Function(Pointer<Void>)>('Paragraph::maxIntrinsicWidth', isLeaf: true)
+ @Native<Double Function(Pointer<Void>)>(symbol: 'Paragraph::maxIntrinsicWidth', isLeaf: true)
external double get maxIntrinsicWidth;
/// The distance from the top of the paragraph to the alphabetic
/// baseline of the first line, in logical pixels.
- @FfiNative<Double Function(Pointer<Void>)>('Paragraph::alphabeticBaseline', isLeaf: true)
+ @Native<Double Function(Pointer<Void>)>(symbol: 'Paragraph::alphabeticBaseline', isLeaf: true)
external double get alphabeticBaseline;
/// The distance from the top of the paragraph to the ideographic
/// baseline of the first line, in logical pixels.
- @FfiNative<Double Function(Pointer<Void>)>('Paragraph::ideographicBaseline', isLeaf: true)
+ @Native<Double Function(Pointer<Void>)>(symbol: 'Paragraph::ideographicBaseline', isLeaf: true)
external double get ideographicBaseline;
/// True if there is more vertical content, but the text was truncated, either
@@ -2744,7 +2744,7 @@
///
/// See the discussion of the `maxLines` and `ellipsis` arguments at
/// [ParagraphStyle.new].
- @FfiNative<Bool Function(Pointer<Void>)>('Paragraph::didExceedMaxLines', isLeaf: true)
+ @Native<Bool Function(Pointer<Void>)>(symbol: 'Paragraph::didExceedMaxLines', isLeaf: true)
external bool get didExceedMaxLines;
/// Computes the size and position of each glyph in the paragraph.
@@ -2757,7 +2757,7 @@
return true;
}());
}
- @FfiNative<Void Function(Pointer<Void>, Double)>('Paragraph::layout', isLeaf: true)
+ @Native<Void Function(Pointer<Void>, Double)>(symbol: 'Paragraph::layout', isLeaf: true)
external void _layout(double width);
List<TextBox> _decodeTextBoxes(Float32List encoded) {
@@ -2796,7 +2796,7 @@
}
// See paragraph.cc for the layout of this return value.
- @FfiNative<Handle Function(Pointer<Void>, Uint32, Uint32, Uint32, Uint32)>('Paragraph::getRectsForRange')
+ @Native<Handle Function(Pointer<Void>, Uint32, Uint32, Uint32, Uint32)>(symbol: 'Paragraph::getRectsForRange')
external Float32List _getBoxesForRange(int start, int end, int boxHeightStyle, int boxWidthStyle);
/// Returns a list of text boxes that enclose all placeholders in the paragraph.
@@ -2810,7 +2810,7 @@
return _decodeTextBoxes(_getBoxesForPlaceholders());
}
- @FfiNative<Handle Function(Pointer<Void>)>('Paragraph::getRectsForPlaceholders')
+ @Native<Handle Function(Pointer<Void>)>(symbol: 'Paragraph::getRectsForPlaceholders')
external Float32List _getBoxesForPlaceholders();
/// Returns the text position closest to the given offset.
@@ -2819,7 +2819,7 @@
return TextPosition(offset: encoded[0], affinity: TextAffinity.values[encoded[1]]);
}
- @FfiNative<Handle Function(Pointer<Void>, Double, Double)>('Paragraph::getPositionForOffset')
+ @Native<Handle Function(Pointer<Void>, Double, Double)>(symbol: 'Paragraph::getPositionForOffset')
external List<int> _getPositionForOffset(double dx, double dy);
/// Returns the [TextRange] of the word at the given [TextPosition].
@@ -2848,7 +2848,7 @@
return TextRange(start: boundary[0], end: boundary[1]);
}
- @FfiNative<Handle Function(Pointer<Void>, Uint32)>('Paragraph::getWordBoundary')
+ @Native<Handle Function(Pointer<Void>, Uint32)>(symbol: 'Paragraph::getWordBoundary')
external List<int> _getWordBoundary(int offset);
/// Returns the [TextRange] of the line at the given [TextPosition].
@@ -2880,13 +2880,13 @@
return line;
}
- @FfiNative<Handle Function(Pointer<Void>, Uint32)>('Paragraph::getLineBoundary')
+ @Native<Handle Function(Pointer<Void>, Uint32)>(symbol: 'Paragraph::getLineBoundary')
external List<int> _getLineBoundary(int offset);
// Redirecting the paint function in this way solves some dependency problems
// in the C++ code. If we straighten out the C++ dependencies, we can remove
// this indirection.
- @FfiNative<Void Function(Pointer<Void>, Pointer<Void>, Double, Double)>('Paragraph::paint')
+ @Native<Void Function(Pointer<Void>, Pointer<Void>, Double, Double)>(symbol: 'Paragraph::paint')
external void _paint(Canvas canvas, double x, double y);
/// Returns the full list of [LineMetrics] that describe in detail the various
@@ -2917,7 +2917,7 @@
return metrics;
}
- @FfiNative<Handle Function(Pointer<Void>)>('Paragraph::computeLineMetrics')
+ @Native<Handle Function(Pointer<Void>)>(symbol: 'Paragraph::computeLineMetrics')
external Float64List _computeLineMetrics();
/// Release the resources used by this object. The object is no longer usable
@@ -2933,7 +2933,7 @@
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
- @FfiNative<Void Function(Pointer<Void>)>('Paragraph::dispose')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'Paragraph::dispose')
external void _dispose();
bool _disposed = false;
@@ -3004,7 +3004,7 @@
);
}
- @FfiNative<Void Function(Handle, Handle, Handle, Handle, Handle, Double, Double, Handle, Handle)>('ParagraphBuilder::Create')
+ @Native<Void Function(Handle, Handle, Handle, Handle, Handle, Double, Double, Handle, Handle)>(symbol: 'ParagraphBuilder::Create')
external void _constructor(
Int32List encoded,
ByteData? strutData,
@@ -3084,7 +3084,7 @@
);
}
- @FfiNative<
+ @Native<
Void Function(
Pointer<Void>,
Handle,
@@ -3101,7 +3101,7 @@
Handle,
Handle,
Handle,
- Handle)>('ParagraphBuilder::pushStyle')
+ Handle)>(symbol: 'ParagraphBuilder::pushStyle')
external void _pushStyle(
Int32List encoded,
List<Object?> fontFamilies,
@@ -3128,7 +3128,7 @@
/// added to the paragraph is affected by all the styles in the stack. Calling
/// [pop] removes the topmost style in the stack, leaving the remaining styles
/// in effect.
- @FfiNative<Void Function(Pointer<Void>)>('ParagraphBuilder::pop', isLeaf: true)
+ @Native<Void Function(Pointer<Void>)>(symbol: 'ParagraphBuilder::pop', isLeaf: true)
external void pop();
/// Adds the given text to the paragraph.
@@ -3141,7 +3141,7 @@
}
}
- @FfiNative<Handle Function(Pointer<Void>, Handle)>('ParagraphBuilder::addText')
+ @Native<Handle Function(Pointer<Void>, Handle)>(symbol: 'ParagraphBuilder::addText')
external String? _addText(String text);
/// Adds an inline placeholder space to the paragraph.
@@ -3208,7 +3208,7 @@
_placeholderScales.add(scale);
}
- @FfiNative<Void Function(Pointer<Void>, Double, Double, Uint32, Double, Uint32)>('ParagraphBuilder::addPlaceholder')
+ @Native<Void Function(Pointer<Void>, Double, Double, Uint32, Double, Uint32)>(symbol: 'ParagraphBuilder::addPlaceholder')
external void _addPlaceholder(double width, double height, int alignment, double baselineOffset, int baseline);
/// Applies the given paragraph style and returns a [Paragraph] containing the
@@ -3222,7 +3222,7 @@
return paragraph;
}
- @FfiNative<Void Function(Pointer<Void>, Handle)>('ParagraphBuilder::build')
+ @Native<Void Function(Pointer<Void>, Handle)>(symbol: 'ParagraphBuilder::build')
external void _build(Paragraph outParagraph);
}
@@ -3259,5 +3259,5 @@
}
}
-@FfiNative<Void Function(Handle, Handle, Handle)>('FontCollection::LoadFontFromList')
+@Native<Void Function(Handle, Handle, Handle)>(symbol: 'FontCollection::LoadFontFromList')
external void _loadFontFromList(Uint8List list, _Callback<void> callback, String fontFamily);
diff --git a/lib/ui/window.dart b/lib/ui/window.dart
index b823dd3..e37a485 100644
--- a/lib/ui/window.dart
+++ b/lib/ui/window.dart
@@ -267,7 +267,7 @@
/// painting.
void render(Scene scene) => _render(scene);
- @FfiNative<Void Function(Pointer<Void>)>('PlatformConfigurationNativeApi::Render')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'PlatformConfigurationNativeApi::Render')
external static void _render(Scene scene);
/// Change the retained semantics data about this [FlutterView].
@@ -280,7 +280,7 @@
/// cannot be used further.
void updateSemantics(SemanticsUpdate update) => _updateSemantics(update);
- @FfiNative<Void Function(Pointer<Void>)>('PlatformConfigurationNativeApi::UpdateSemantics')
+ @Native<Void Function(Pointer<Void>)>(symbol: 'PlatformConfigurationNativeApi::UpdateSemantics')
external static void _updateSemantics(SemanticsUpdate update);
}
diff --git a/third_party/tonic/tests/fixtures/tonic_test.dart b/third_party/tonic/tests/fixtures/tonic_test.dart
index 38c5fb0..4d408fd 100644
--- a/third_party/tonic/tests/fixtures/tonic_test.dart
+++ b/third_party/tonic/tests/fixtures/tonic_test.dart
@@ -32,7 +32,7 @@
// Test helpers for simple void calls through Tonic.
-@FfiNative<Void Function()>('Nop', isLeaf: true)
+@Native<Void Function()>(symbol: 'Nop', isLeaf: true)
external void nop();
@pragma('vm:entry-point')
@@ -43,7 +43,7 @@
// Test helpers for calls with bool through Tonic.
-@FfiNative<Bool Function(Bool)>('EchoBool')
+@Native<Bool Function(Bool)>(symbol: 'EchoBool')
external bool echoBool(bool arg);
@pragma('vm:entry-point')
@@ -55,7 +55,7 @@
// Test helpers for calls with int through Tonic.
-@FfiNative<IntPtr Function(IntPtr)>('EchoIntPtr')
+@Native<IntPtr Function(IntPtr)>(symbol: 'EchoIntPtr')
external int echoIntPtr(int arg);
@pragma('vm:entry-point')
@@ -67,7 +67,7 @@
// Test helpers for calls with float through Tonic.
-@FfiNative<Float Function(Float)>('EchoFloat')
+@Native<Float Function(Float)>(symbol: 'EchoFloat')
external double echoFloat(double arg);
@pragma('vm:entry-point')
@@ -79,7 +79,7 @@
// Test helpers for calls with double through Tonic.
-@FfiNative<Double Function(Double)>('EchoDouble')
+@Native<Double Function(Double)>(symbol: 'EchoDouble')
external double echoDouble(double arg);
@pragma('vm:entry-point')
@@ -91,7 +91,7 @@
// Test helpers for calls with Dart_Handle through Tonic.
-@FfiNative<Handle Function(Handle)>('EchoHandle')
+@Native<Handle Function(Handle)>(symbol: 'EchoHandle')
external Object echoHandle(Object arg);
@pragma('vm:entry-point')
@@ -103,7 +103,7 @@
// Test helpers for calls with std::string through Tonic.
-@FfiNative<Handle Function(Handle)>('EchoString')
+@Native<Handle Function(Handle)>(symbol: 'EchoString')
external String echoString(String arg);
@pragma('vm:entry-point')
@@ -115,7 +115,7 @@
// Test helpers for calls with std::u16string through Tonic.
-@FfiNative<Handle Function(Handle)>('EchoU16String')
+@Native<Handle Function(Handle)>(symbol: 'EchoU16String')
external String echoU16String(String arg);
@pragma('vm:entry-point')
@@ -127,7 +127,7 @@
// Test helpers for calls with std::vector through Tonic.
-@FfiNative<Handle Function(Handle)>('EchoVector')
+@Native<Handle Function(Handle)>(symbol: 'EchoVector')
external List<String> echoVector(List<String> arg);
@pragma('vm:entry-point')
@@ -144,19 +144,17 @@
_Create(this, value);
}
- @FfiNative<Void Function(Handle, IntPtr)>('CreateNative')
+ @Native<Void Function(Handle, IntPtr)>(symbol: 'CreateNative')
external static void _Create(MyNativeClass self, int value);
- @FfiNative<Int32 Function(Pointer<Void>, Int32, Handle)>(
- 'MyNativeClass::MyTestFunction')
+ @Native<Int32 Function(Pointer<Void>, Int32, Handle)>(symbol: 'MyNativeClass::MyTestFunction')
external static int myTestFunction(MyNativeClass self, int x, Object handle);
- @FfiNative<Handle Function(Pointer<Void>, Int64)>(
- 'MyNativeClass::MyTestMethod')
+ @Native<Handle Function(Pointer<Void>, Int64)>(symbol: 'MyNativeClass::MyTestMethod')
external Object myTestMethod(int a);
}
-@FfiNative<IntPtr Function(Pointer<Void>)>('EchoWrappable')
+@Native<IntPtr Function(Pointer<Void>)>(symbol: 'EchoWrappable')
external int echoWrappable(MyNativeClass arg);
@pragma('vm:entry-point')
@@ -169,7 +167,7 @@
// Test helpers for calls with TypedList<..> through Tonic.
-@FfiNative<Handle Function(Handle)>('EchoTypedList')
+@Native<Handle Function(Handle)>(symbol: 'EchoTypedList')
external Float32List echoTypedList(Float32List arg);
@pragma('vm:entry-point')